What is the simplest C# code to copy directory? In the last post, I mentioned writing an automated tool software in today’s work. One of the functions is to copy directories. At the beginning, I used recursive code to copy directories and found that its work efficiency is very low. So it took some time to find the simplest and most efficient code.
Simplest C# code to copy directory
Like most programmers, I started out using the standard code provided by Microsoft’s official documentation for directory copying.
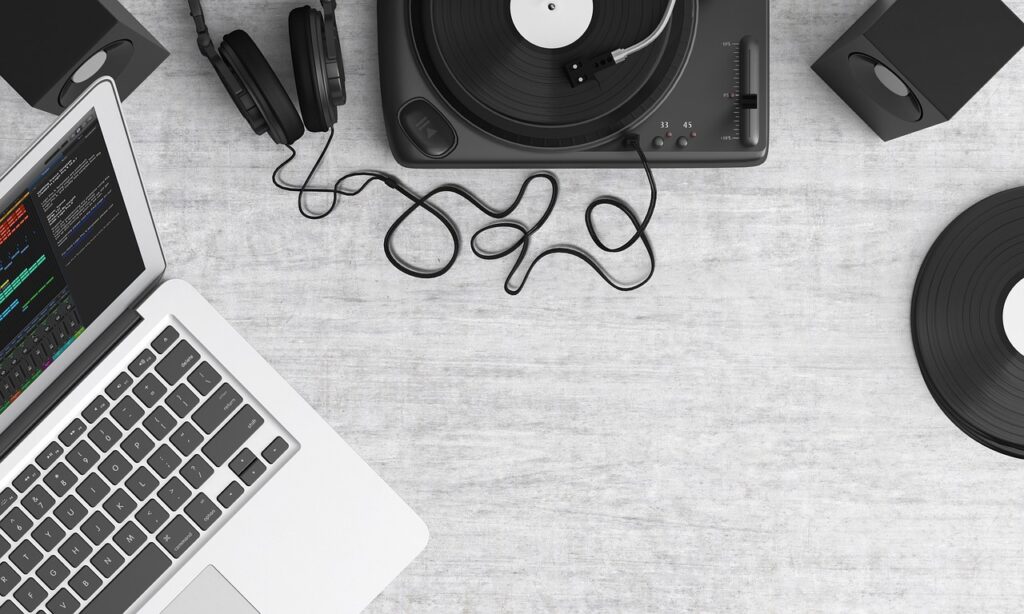
Code from: https://docs.microsoft.com/en-us/dotnet/standard/io/how-to-copy-directories
Initial copy directory code
using System.IO; CopyDirectory(@".\", @".\copytest", true); static void CopyDirectory(string sourceDir, string destinationDir, bool recursive) { // Get information about the source directory var dir = new DirectoryInfo(sourceDir); // Check if the source directory exists if (!dir.Exists) throw new DirectoryNotFoundException($"Source directory not found: {dir.FullName}"); // Cache directories before we start copying DirectoryInfo[] dirs = dir.GetDirectories(); // Create the destination directory Directory.CreateDirectory(destinationDir); // Get the files in the source directory and copy to the destination directory foreach (FileInfo file in dir.GetFiles()) { string targetFilePath = Path.Combine(destinationDir, file.Name); file.CopyTo(targetFilePath); } // If recursive and copying subdirectories, recursively call this method if (recursive) { foreach (DirectoryInfo subDir in dirs) { string newDestinationDir = Path.Combine(destinationDir, subDir.Name); CopyDirectory(subDir.FullName, newDestinationDir, true); } } }
What is the simplest C# code to copy directory?
This code doesn’t work very well when it’s actually used in your production. I have 39 directories, and each directory has many subdirectories nested inside, and each directory has many code files, in fact, they are the source code of the asp.net webform website, you know, a site hundreds of Pages are very common, and the total file size of these 39 directories is about 3GB.
Even using the copy function on the computer, it would take about 10 minutes to copy these 39 directories to another directory.
Copy a directory with Xcopy
Since I can’t bear the above code to work too slowly, I use Xcopy to refactor the code, you only need to encapsulate a C# auxiliary class that runs cmd, and then copy the directory through Xcopy.
In the previous post, I have given a Cmd Executor class, which can be very convenient to use to copy directories. code show as below:
Task.Factory.StartNew(() => { string cmd = $@"Xcopy /E /I {item.FullDirectoryPath} {Path.Combine(textBox1.Text, new DirectoryInfo(item.FullDirectoryPath).Name)}"; CmdExecutor.ExecuteCmd(new string[] { cmd }, ".", (a, b) => ShowLog(b.Data)); });
What is the simplest C# code to copy directory?
In the real code, I put it in the Task to run, you can notice that the Xcopy code above plays a key role.
Task.Factory.StartNew(() => { string destinationDir = Path.Combine(textBox1.Text, new DirectoryInfo(item.FullDirectoryPath).Name); ShowLog($"Start copying the source site directory:{item.FullDirectoryPath} to the target directory:{destinationDir}"); FileSystem.CopyDirectory(item.FullDirectoryPath, Path.Combine(textBox1.Text, new DirectoryInfo(item.FullDirectoryPath).Name)); ShowLog($"File copy completed:{item.FullDirectoryPath} to the target directory:{destinationDir}"); });
What is the simplest C# code to copy directory?
The above piece of code has been actually tested, and the efficiency is higher than the Initial copy directory code, but the disadvantage is that it needs to start an additional cmd process, if only functionally, it is enough.
Is there an easier way then? The answer is, yes.
Only one line of code
What I believe in is that the simpler it is, the less likely it is to make mistakes. Of course, there is a logic behind the replication hidden behind the simplicity.
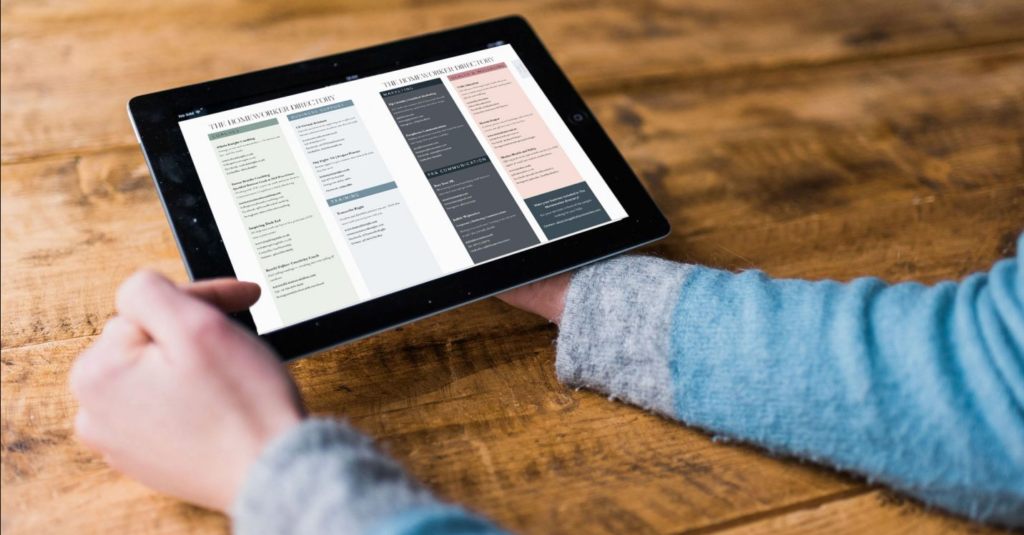
In the end my code for copying a directory using C# looks like this, as you can see, it only takes one line of code to work.
foreach (var item in sources) { Task.Factory.StartNew(() => { string destinationDir = Path.Combine(textBox1.Text, new DirectoryInfo(item.FullDirectoryPath).Name); FileSystem.CopyDirectory(item.FullDirectoryPath, Path.Combine(textBox1.Text, new DirectoryInfo(item.FullDirectoryPath).Name)); }); }
What is the simplest C# code to copy directory?
The core of the code snippet provided above is to call the FileSystem.CopyDirectory method. Its namespace is: Microsoft.VisualBasic.FileIO, so you need to add a reference to call this method.
Conclusion
When you are writing C# code to copy directories, you may copy the code recursively, as I did in the original version, and either way, you can complete the function of copying directories.
But the crux of the matter is that when the size of the directory you need to copy is large, and given the principle of simplicity, I prefer to use the version that ends up being one line of code to copy the directory, on a production server, it works well and is efficient very high.